Launching Wordpress over Kubernetes and connecting the same with MySQL database over AWS RDS

Problem Statement
Deploy the Wordpress application on Kubernetes and AWS using terraform including the following steps;
1. Write an Infrastructure as code using terraform, which automatically deploy the Wordpress application
2. On AWS, use RDS service for the relational database for Wordpress application.
3. Deploy the Wordpress as a container either on top of Minikube or EKS or Fargate service on AWS.
4. The Wordpress application should be accessible from the public world if deployed on AWS or through workstation if deployed on Minikube.
Try each step first manually and write Terraform code for the same to have a proper understanding of workflow of task.
Solution
Step 1: The following code briefs about the providers in this code i.e. Kubernetes and AWS.
provider "kubernetes"{ config_context_cluster="minikube"}
provider "aws"{ region="ap-south-1" profile="rkd"}
Step 2: We now bring our default VPCs and subnets in action rather than creating a new one everytime.
data "aws_vpc" "def_vpc"{ default=true}data "aws_subnet_ids" "vpc_sub"{ vpc_id = data.aws_vpc.def_vpc.id}
Step 3: Creating the security groups for our SQL database with ingress of port 3306 as SQL works on the same.
resource "aws_security_group" "allow_sql" { name = "mydb-sg" description = "Allow sql" vpc_id = data.aws_vpc.def_vpc.id ingress { description = "for sql" from_port = 3306 to_port = 3306 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } egress { from_port = 0 to_port = 0 protocol = "-1" cidr_blocks = ["0.0.0.0/0"] } tags = { Name = "mydb-sg" }}
Step 4: Creating a subnet group for the database instance.
resource "aws_db_subnet_group" "sub_ids" { name = "main" subnet_ids = data.aws_subnet_ids.vpc_sub.ids tags = { Name = "DB subnet group" }}
Step 5: Creating a database instance with its proper configurations
resource "aws_db_instance" "my-db" { allocated_storage = 20 storage_type = "gp2" engine = "mysql" engine_version = "5.7" instance_class = "db.t2.micro" name = "mydb" username = "<your username>" password = "<your password>" parameter_group_name = "default.mysql5.7" db_subnet_group_name = aws_db_subnet_group.sub_ids.id vpc_security_group_ids = [aws_security_group.allow_sql.id] publicly_accessible = true skip_final_snapshot = true}
Step 6: Creating a K8s deployment which deploys Wordpress over minikube.
resource "kubernetes_deployment" "wp-deploy"{ depends_on = [ aws_db_instance.my-db ] metadata{ name="wordpress-deploy" } spec{ replicas=1 selector{ match_labels={ env="production" region="IN" app="wordpress" } } template{ metadata { labels={ env="production" region="IN" app="wordpress" } } spec{ container{ image="wordpress:4.8-apache" name="mywp-con" env { name = "WORDPRESS_DB_HOST" value = aws_db_instance.my-db.endpoint } env { name = "WORDPRESS_DB_DATABASE" value = aws_db_instance.my-db.name } env { name = "WORDPRESS_DB_USER" value = aws_db_instance.my-db.username } env { name = "WORDPRESS_DB_PASSWORD" value = aws_db_instance.my-db.password } port { container_port = 80 } } } } }}
Step 7: Creating a load balancer to manage the traffic.
resource "kubernetes_service" "wordpress"{ depends_on = [ kubernetes_deployment.wp-deploy, ] metadata{ name="wordpress" } spec{ selector={ app = "wordpress" } port{ node_port=31002 port=80 target_port=80 } type="NodePort" }}
Step 8: Creating a null resource which automatically opens chrome for the output.
resource "null_resource" "openwebsite" { depends_on = [ kubernetes_service.wordpress ] provisioner "local-exec" { command = "minikube service ${kubernetes_service.wordpress.metadata[0].name}" }}
Start your minikube with the following command.
minikube start
The minikube gets triggered as shown.
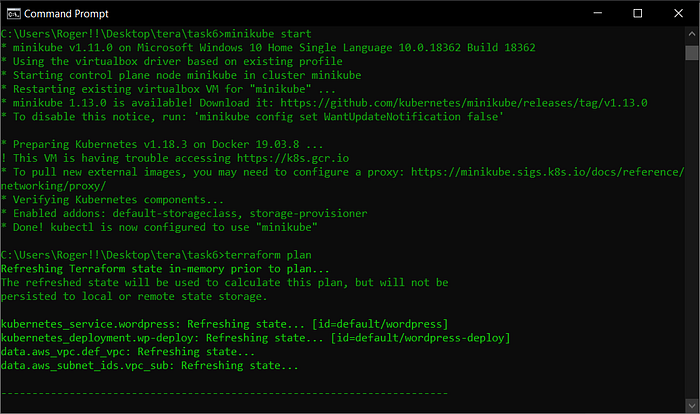
Run the following command to download the required plugins
terraform init
Use the following command to get the blueprint of what the code is going to do to the machine.
terraform plan
And finally use this command to execute the code.
terraform apply --auto-approve
The process begins…


The Chrome browser opens automatically!



Following is the database launched in AWS RDS.


To check the pods launched over Kubernetes use the following command.
kubectl get all

Hence the Wordpress launched over kubernetes is now connected with the database which was over AWS RDS
To delete the entire set up use the following command.
terraform destroy --auto-approve


To delete setup of Kubernetes use the following command.
kubectl delete all --all

Attaching my GitHub repo here.
Thanks for your time. :)
Happy reading!